Build a Telegram Bot using Laravel Framework
Getting Started with Telegram using Laravel Framework, we will guide you set up a Telegram bot using Laravel Framework.
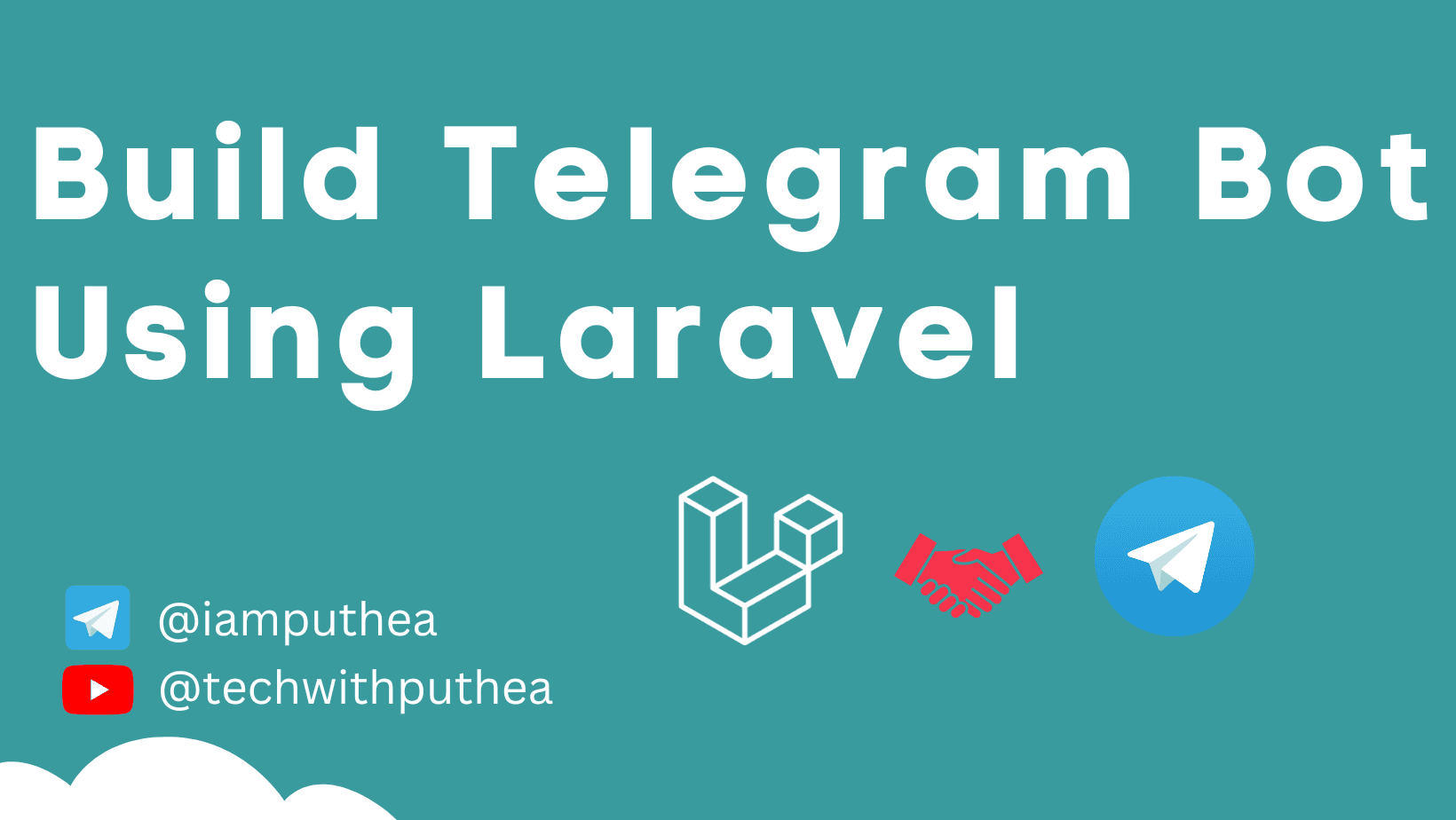
Introduction
Set up Laravel Project
Suppose you have already installed Laravel on your machine. If not, you can follow the official documentation to install Laravel.
composer create-project --prefer-dist laravel/laravel telegram-bot
or
laravel new telegram-bot
Create Telegram Bot
To create a Telegram bot, you need to create a new bot using the BotFather. Open your Telegram app and search for BotFather. Click on the BotFather and type /newbot
. Follow the instructions to create a new bot.
Set up Laravel Bot
Once you have created a new bot, you will get a token. Copy the token and save it somewhere safe. Now, let's set up the Laravel bot.
composer require irazasyed/telegram-bot-sdk
Configure Telegram Bot SDK
Add the following configuration to the .env
file.
TELEGRAM_BOT_TOKEN=YOUR_BOT_TOKEN
TELEGRAM_BOT_USERNAME=YOUR_BOT_USERNAME
YOUR_BOT_TOKEN
A token Generate from BotFather.
Publish the Configuration File
php artisan vendor:publish --provider="Telegram\Bot\Laravel\TelegramServiceProvider"
Create a Controller
You can handle bot updates by creating a controller. Use this command to generate a controller:
php artisan make:controller TelegramController
Inside the controller, you can define the logic to handle Telegram bot updates, such as responding to commands:
<?php
namespace App\Http\Controllers;
use Telegram\Bot\Api;
use Illuminate\Http\Request;
class TelegramBotController extends Controller
{
// Telegram API instance
protected $telegram;
// Constructor to initialize the Telegram Bot API with the token from the .env file
public function __construct()
{
$this->telegram = new Api(env('TELEGRAM_BOT_TOKEN'));
}
// Method to handle incoming webhook requests from Telegram
public function webhook(Request $request)
{
// Get the latest update sent by Telegram via the webhook
$updates = $this->telegram->getWebhookUpdates();
// Extract the message object from the updates
$message = $updates->getMessage();
// Get the text of the message sent by the user
$text = $message->getText();
// Get the unique chat ID where the message was sent from
$chatId = $message->getChat()->getId();
// If the message text is '/start', send a welcome message back to the user
if ($text === '/start') {
$response = "Welcome to the Laravel Telegram Bot!";
// Use the Telegram API to send the response message to the same chat
$this->telegram->sendMessage([
'chat_id' => $chatId, // The ID of the chat to send the message to
'text' => $response // The message text to send
]);
}
}
}
Set up Webhook
To receive updates from Telegram, you need to set up a webhook. Create a route for the bot to listen to:
Route::post('/webhook', [TelegramBotController::class, 'webhook']);
You also need to set the webhook URL in Telegram using this code:
php artisan tinker
// Then run:
Telegram::setWebhook(['url' => 'https://your-app-url/webhook']);
Replace https://your-app-url/webhook with the actual URL of your Laravel app. Ensure the URL is served over HTTPS, as Telegram requires it for webhooks.
Test the Bot
Send /start
to your bot, and it should respond with "Welcome to the Laravel Telegram Bot!"
That's it. If you have any problem contact me t.me/iamputhea