How to Configure Minio as an S3-Compatible Storage in Laravel
Learn how to configure Minio as an S3-compatible storage solution in a Laravel project using Laravel Sail. This guide walks you through setting up Minio, configuring Laravel's filesystem, and storing files with Laravel's storage facade.
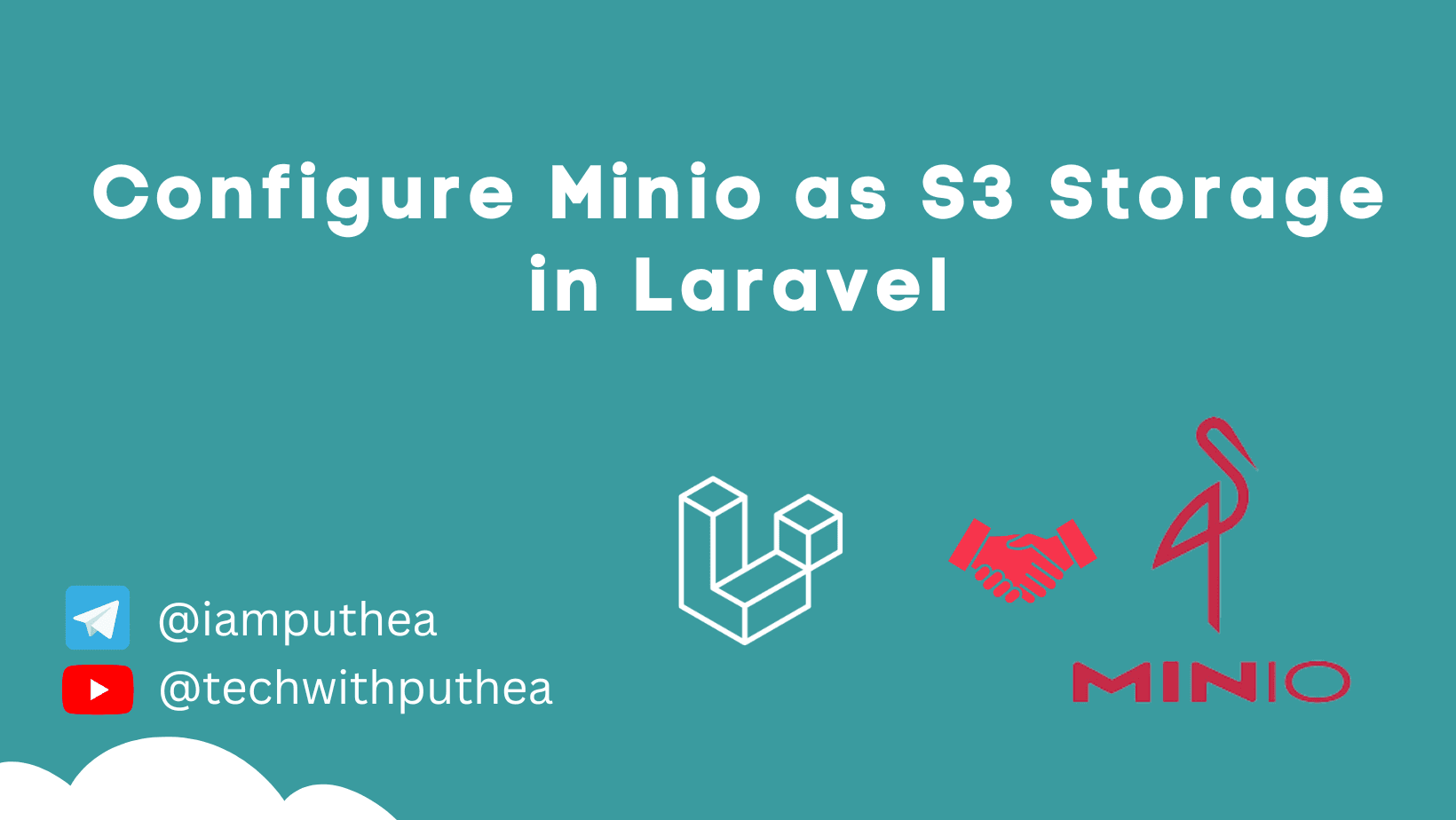
Prerequisites
Before starting, ensure you have the following installed:
- Docker
- Laravel Sail installed in your project
- Basic knowledge of Laravel's filesystem
Step 1: Add Minio to Laravel Sail
Modify your docker-compose.yml
file inside your Laravel project to include Minio:
services:
minio:
image: minio/minio
ports:
- "9000:9000"
- "9001:9001"
environment:
MINIO_ROOT_USER: minioadmin
MINIO_ROOT_PASSWORD: minioadmin
command: server --console-address ":9001" /data
volumes:
- minio-data:/data
volumes:
minio-data:
This configuration starts a Minio instance accessible at http://localhost:9001
(web console) and http://localhost:9000
(API).
Step 2: Configure Laravel to Use Minio
Update your .env
file with Minio credentials:
AWS_ACCESS_KEY_ID=minioadmin
AWS_SECRET_ACCESS_KEY=minioadmin
AWS_DEFAULT_REGION=us-east-1
AWS_BUCKET=laravel-bucket
AWS_ENDPOINT=http://minio:9000
AWS_USE_PATH_STYLE_ENDPOINT=true
Modify config/filesystems.php
to support Minio:
's3' => [
'driver' => 's3',
'key' => env('AWS_ACCESS_KEY_ID'),
'secret' => env('AWS_SECRET_ACCESS_KEY'),
'region' => env('AWS_DEFAULT_REGION'),
'bucket' => env('AWS_BUCKET'),
'url' => env('AWS_URL'),
'endpoint' => env('AWS_ENDPOINT'),
'use_path_style_endpoint' => env('AWS_USE_PATH_STYLE_ENDPOINT', true),
],
Step 3: Start Laravel Sail and Minio
Run the following command to start Sail with Minio:
sail up -d
Then, create a bucket in Minio using the web UI at http://localhost:9001
or using the Minio CLI:
sail minio-client alias set local http://minio:9000 minioadmin minioadmin
sail minio-client mb local/laravel-bucket
Step 4: Store and Retrieve Files
Use Laravel’s Storage facade to interact with Minio:
use Illuminate\Support\Facades\Storage;
// Store a file
Storage::disk('s3')->put('example.txt', 'Hello, Minio!');
// Retrieve a file
$content = Storage::disk('s3')->get('example.txt');
// Generate a temporary URL
$url = Storage::disk('s3')->temporaryUrl('example.txt', now()->addMinutes(5));
Troubleshooting
- If Minio is not reachable, ensure the service is running using
sail ps
. - If files are not being stored, check if the bucket exists using
sail minio-client ls local
. - Ensure
AWS_USE_PATH_STYLE_ENDPOINT=true
in.env
to avoid S3 signature issues.
That’s it! You now have Minio configured as an S3-compatible storage in Laravel using Sail.
If you have any questions, contact me on Telegram.