Laravel 11 Generics Notations for Eloquent Relationships
Laravel introduces generics notations to enhance type safety with PHPStan and IDE support and one of them is relations
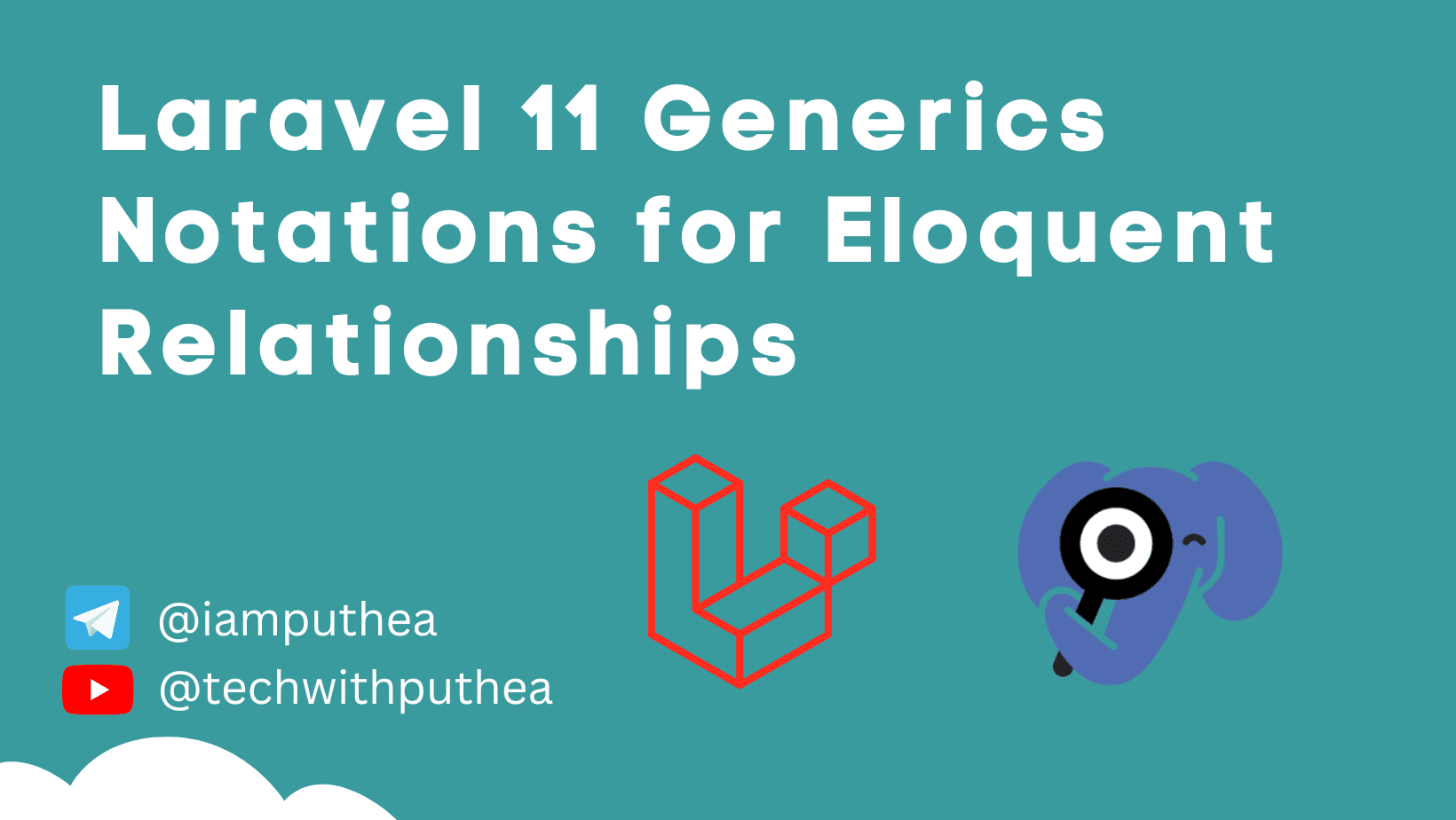
Benefits of Using Generics
- Better Auto-completion: IDEs like PhpStorm can provide more accurate suggestions when navigating relationships.
- Improved Type Safety: You’ll catch errors earlier, such as trying to use an incompatible model in a relationship.
- Cleaner Code: It makes relationships more self-explanatory by clearly showing the types of related models.
HasOne and HasMany
For hasOne and hasMany relationships, you can specify the type of the related model:
/**
* @return HasOne<Profile>
*/
public function profile()
{
return $this->hasOne(Profile:class);
}
/**
* @return HasMany<Comment>
*/
public function comments()
{
return $this->hasMany(Comment:class);
}
BelongsTo and BelongsToMany
For BelongsTo and belongsToMany relationships, you can specify the type of the related model:
/**
* @return BelongsTo<LawLevel, RegulatorySandbox>
*/
public function lawLevel(): BelongsTo
{
return $this->belongsTo(LawLevel::class);
}
/**
* @return BelongsToMany<Role>
*/
public function roles()
{
return $this->belongsToMany(Role::class);
}
HasOneThrough and HasManyThrough
/**
* @return HasManyThrough<Post>
*/
public function posts()
{
return $this->hasManyThrough(Post::class, User::class);
}
/**
* @return HasOneThrough<Post>
*/
public function postThrough()
{
return $this->hasOneThrough(Post::class, User::class);
}
MorphTo and MorphOne
/**
* @return MorphTo<Model, Image>
*/
public function imageable()
{
return $this->morphTo();
}
/**
* @return MorphOne<Image>
*/
public function image()
{
return $this->morphOne(Image::class, 'imageable');
}
MorphMany and MorphToMany
/**
* @return MorphMany<Comment>
*/
public function comments()
{
return $this->morphMany(Comment::class, 'commentable);
}
/**
* @return MorphToMany<Tag>
*/
public function tags()
{
return $this->morphToMany(Tag::class, 'taggable');
}
Work with Factory
class RegulatorySandbox extends Model
{
/** @use HasFactory<RegulatorySandboxFactory> */
use HasFactory;
}
/**
* @extends Factory<RegulatorySandbox>
*/
class RegulatorySandboxFactory extends Factory
{
}
Laravel's support for generics in relationships brings precision, improved IDE hints, and stronger type safety to your codebase. By using these notations, you reduce errors and increase the readability of your relationships.
That's it. If you have any problem contact me t.me/iamputhea